Overview of Conditionals, Loops and Motion
The first programs in this course were simple. Each program was divided into a specified sequence of steps, and each step was completely determined.
-
In converting quarts to liters, the user entered a value, a computation followed a well-known, given formula, and a result was printed.
-
After eSpeak was started, the spoken narrative followed a pre-determined script.
-
The robot beeped a specific tune and/or moved in a prescribed manner.
Although these programs addressed specified tasks, the programs had very limited scope, and more complicated problems require additional processing capabilities.
Module Goals
This module explores in some detail several basic elements of problem solving with C. Through this study, students will learn how to
-
declare variables of different types and distinguish local from global variables,
-
specify the output format for integers, real numbers, and identifying text,
-
instruct a program to behave in alternative ways, depending upon identified circumstances,
-
repeat a sequence of instructions within a program, and
-
control robot behavior, based on data from various sensors.
Example: Maze running
Consider the challenge of moving a robot through a maze. That is, the robot is placed within an environment with walls, and the robot must find a path that leads out of the maze.
One simple algorithm involves moving a robot forward. Once an obstacle is encountered, the robot turns right (until no obstacle is detected) and continues moving forward. This process of moving forward and turning right continues until the maze is left behind.
This algorithm works in some cases (such as the example at the right), but it may not be very efficient. The reader is encouraged to develop a more sophisticated algorithm that keeps track of where the robot has already been (to avoid moving in circles) and that turns right or left through openings in the middle of a long run forward.
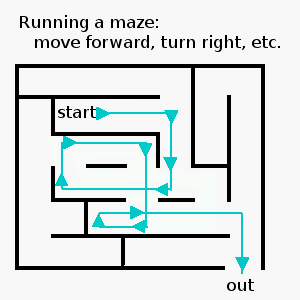
Example: Moving a robot along a path
Suppose a robot is to be directed to move along a path until it reaches a destination.
For this problem, the robot might take a picture of the path ahead, locate the edges of the path, and move forward, left, or right as the path continues straight or turns.
Challenges: Making decisions and repeating actions
Each of the examples (maze running and following a path) require the robot to move, based on current information about its surroundings. In moving through a maze, the robot may move forward until it encounters an obstacle, but the program cannot anticipate where the wall might be. Rather, the robot must check for an obstacle as it moves. In following a path, the robot will need to take a picture (or use its sensors), determine the current direction of the path (straight ahead, turning left or right), and move as the path proceeds.
These examples illustrate two vital needs for problem solving, beyond the capabilities illustrated in the programs of the introductory module:
-
A program must be able to make decisions, based on current information. If/when an obstacle is encountered, turn left; if the path turns, make a corresponding turn.
-
A program must be able to repeat a block of instructions. Repeat moving forward and turning right until leaving the maze; continue along the path while taking pictures and turning as appropriate.
Module Overview
The Getting Started module introduced sufficient elements of the C programming language and MyroC to write simple programs. However, the introductory materials could only mention basic syntax (the format of each statement) and semantics (the description of what a program statement means). With the quick introduction now completed, this module explores basic C syntax and semantics more carefully and also expands the discussion to include some additional capabilities. A brief outline follows.
-
Data types and variables: The introductory programs illustrated that C considers integers to be different from real numbers and that each variable must have a stated type (e.g., int or double). Additional discussion is needed to consider the storage of character data and the results obtained when different types are mixed within a single expression.
-
Output format: The quarts-to-liters program allowed the user to enter a number of quarts. After computation, the program printed the corresponding number of liters following a default format. Additional capabilities for printf allow output to be tailored for readability, such as the placement of data within columns and the printing of real numbers with a specified number of decimal places.
-
Conditional statements: C provides two mechanisms (called if and switch) that allow programs to perform different steps, based on current circumstances. For example, after determining whether or not an obstacle is present, a robot might continue straight ahead or turn.
-
Loops: C provides three main mechanisms (called for, while, and do ... while) that allow programs to repeat blocks of instructions.
-
Project: As with all modules in this course, the various elements of this module come together in a concluding module.
Through the module, several examples will explore mechanisms to obtain data from the Scribbler 2 robot's sensors and consider how that information might be used in controlling the robot's behavior.
created 19 April 2016 by Henry M. Walker revised 3 July 2016 by Henry M. Walker |
![]() ![]() |