Goals
In this project, you will implement a variation of the "Pass the Pigs" game. You will first build the core logic for recognizing rolls and scoring, and then write a simple graphical interface to drive the game.
You will be practicing the following concepts from prior labs:
while
-loops, nestedfor
-loops- conditionals
- writing and documenting functions
- lists
- drawing shapes with the graphics package
Summary
"Pass the Pigs" is a dice game played with two, asymmetric, 6-sided dice. The "Pass the Pigs" Wikipedia article has an excellent description of the original game and a photo of the adorable pig dice used in the game.
During the game, two players compete to reach 100 points. Each turn, two dice are thrown at a time. After each roll, a player must decide to either roll again, or hold their score. Due to the asymmetrial nature of the dice, not every throw is equally likely in the game. For example, a pigs lying on its side is very likely, while a pigs landing balanced on a snout and ear (a "leaning jowler") is much rarer.
Part of this project is an exercise in implementing functions to their specification, and matching target outputs. It is not a creative exercise, but rather the opportunity for you to demonstrate understanding of specifications, control over the tools we've learned in the class, and being detail-oriented. You are asked to demonstrate the requested behavior and output, matching both the functions' docstrings and the sample output. In all samples, user input is shown in italics and underlined.
In contrast, the extra credit is a creative exercise, in which you can enhance your user interface in fanciful ways.
Due Dates
- Checkpoint A: Due as a demo in any lab, drop-in tutoring or workshop before Thursday, Mar. 15 at 7 PM.
- Checkpoint B: Due as a demo in any lab, drop-in tutoring or workshop before Sun, Apr. 8 at 7 PM.
- Final Code: Due via Moodle on Thu, Apr. 12 at 11:55 PM.
Template
You will need the graphics package, a helper library and a template for each part of the project:
- Part A: Download the template, template_P2A.py
- Part B: Download the template, template_P2.py
- Part B: Download the helper package, hog_helper.py
- Part B: Download the graphics package, graphics.py
Pig Positions and Scoring
For reference, each pig can land in one of the following 6 positions:
- 'A' = Pig lands on its side (with no dot)
- 'B' = Pig lands on its side (with dot)
- 'C' = Razorback (pig lands on its back)
- 'D' = Trotter (pig is standing upright)
- 'E' = Snouter (pig is leaning on its snout)
- 'F' = Leaning jowler (pig is resting on its snout and ear)
The names and points of all the possible rolls are listed below.
Pig positions | Roll name | Points |
---|---|---|
A, B | Pig Out | 0 |
A, A, or B, B | Sider | 1 |
C, A or C, B | Razorback | 5 |
D, A or D, B | Trotter | 5 |
E, A or E, B | Snouter | 10 |
F, A or F, B | Leaning Jowler | 15 |
C, C | Double Razorback | 20 |
D, D | Double Trotter | 20 |
E, E | Double Snouter | 40 |
F, F | Double Leaning Jowler | 60 |
Any other roll | Mixed combo | Sum of individual rolls |
For example, the roll (C, E) is a mixed combo. It should be scored as a Razorback (C) + a Snouter (E), for a total of 15. Note that it doesn't matter which order the pigs are in.
Checkpoint A
For Checkpoint A, you will need to demonstrate a program that does the following:
- Asks the user for the names of two rolls
- Puts these two strings into a list and prints it.
- Prints the name of the roll.
- Prints the score of the roll.
- If the score is zero, the program ends. Otherwise, repeat this process.
Sample Input/Output
- Sample 1
Enter pig 1: A Enter pig 2: A Faces are ['A', 'A'] Roll is ['Sider'] Score is 1 Enter pig 1: A Enter pig 2: B Faces are ['A', 'B'] Roll is ['Pig Out'] Score is 0
- Sample 2
Enter pig 1: C Enter pig 2: B Faces are ['C', 'B'] Roll is ['Razorback'] Score is 5 Enter pig 1: B Enter pig 2: C Faces are ['B', 'C'] Roll is ['Razorback'] Score is 5 Enter pig 1: E Enter pig 2: C Faces are ['E', 'C'] Roll is ['Razorback', 'Snouter'] Score is 15 Enter pig 1: B Enter pig 2: A Faces are ['B', 'A'] Roll is ['Pig Out'] Score is 0
Checkpoint B
Next, you will evolve your program into a graphical version of the game. Your game is played from the perspective of a single player, letting the user roll the dice until they hit a score of 100.
You need to re-design and expand your program to make use of functions, to demonstrate a program that does the following:
- Download the Part B template and related support files. The template holds specifications for functions you will implement.
- Implement the
name_roll()
andscore_roll()
functions, by refactoring your Checkpoint A logic. The Part B template includes starter code formain()
; when those two functions are properly implemented, the behavior of the code in main will match the functionality of Checkpoint A. - Now, replace
main()
with logic that does the following:- Create a square graphical window of size
WINDOW_SIZE
, using thedraw_main_window()
function. - Create a button in the window, using the
draw_button()
function. - Wait until the user clicks on the button, using the
wait_for_button()
function. - When the button is clicked, use the
roll()
function to get the values of the roll. - Draw two colored squares representing that roll, using the following colors:
- 'A' = red, 'B' = blue, 'C' = yellow, 'D' = green, 'E' = purple, 'F' = orange
- Update the window to draw text for the name of the roll (see hint below).
- Update the window to draw text for the score of the roll (see hint below).
- If the total score is at or above 100, the program ends.
- If the total score is less than 100, repeat the process for the next roll.
Many of the above tasks can be accomplished directly by using the sample code for
main()
, provided below. - Create a square graphical window of size
Demo.Demo Checkpoint B.
Advice and Hints
Hint: To draw text in a graphics window, use the function Text(pt, s)
, where s
is a string that will be centered at Point pt
when drawn.
Here is an example snippet of code that centers the string "Hello" at the point (100,100) in 30-point font:
txt = Text(Point(100,100), "Hello") txt.setSize(30) txt.draw(win)
Hint: It is your responsibility to put appropriate logic into their own functions. The grading rubric requires that you invent and document your own functions. There are many options for this. As inspiration, one possible main()
function is below, highlighting one possible design, to help you organize your own code. You may be able to infer the possible behavior of these functions from this context.
def main(): win = draw_main_window(WINDOW_SIZE) b = draw_button(win, Point(WINDOW_SIZE/2, 50), "ROLL") total = 0 s = display_score(win, total) # draw score (0) n = display_name(win, []) # draw name (blank) wait_for_button(win, b) # get click while (total < 100): s.undraw() # un-draw old score from win n.undraw() # un-draw old name from win d = roll() # roll the dice names = name_roll(d) # get names for roll total += score_roll(names) # sum score for roll display_squares(win, d) # draw squares for roll n = display_name(win, names) # draw name s = display_score(win, total) # draw score wait_for_button(win, b) # wait for next click win.close()
If you cannot intrepret the above code, then it is a worthwhile exercise for you to think through the required behavior of the program on your own and plan out the logic using functions of your own design.
Sample Input/Output
- Sample 3 (Window)
- Sample 4 (Window)
- Sample 5 (Window)
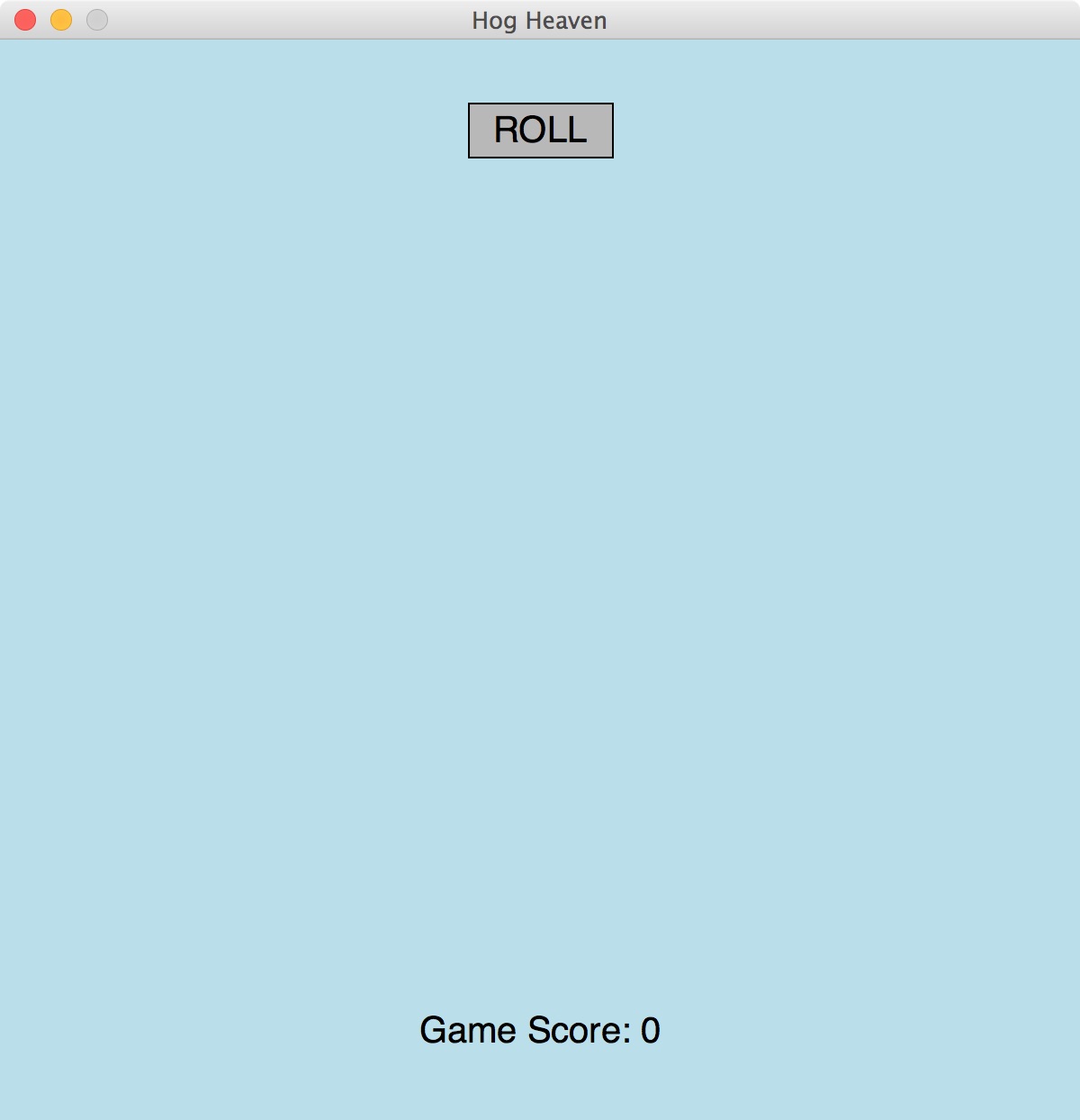
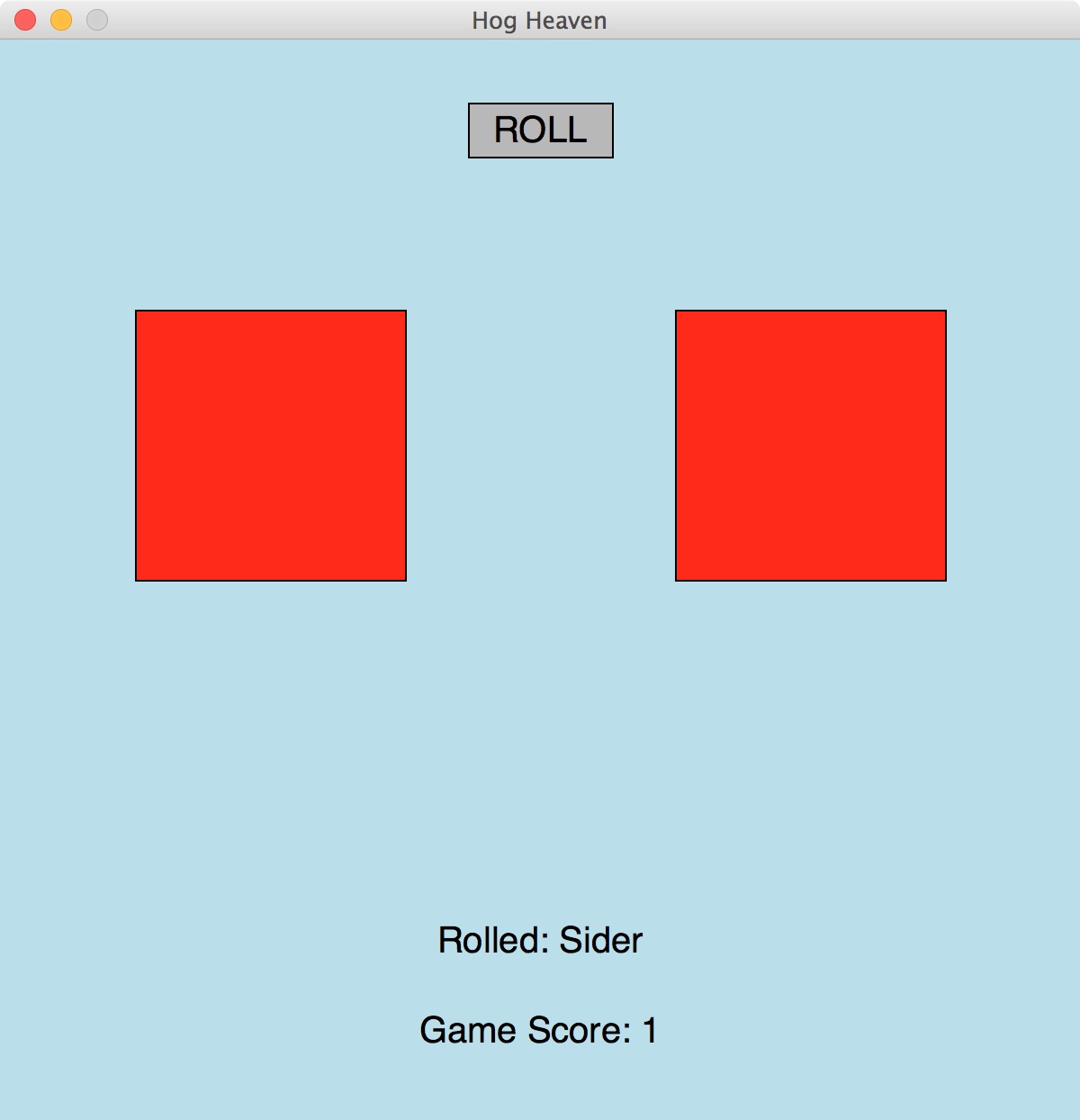
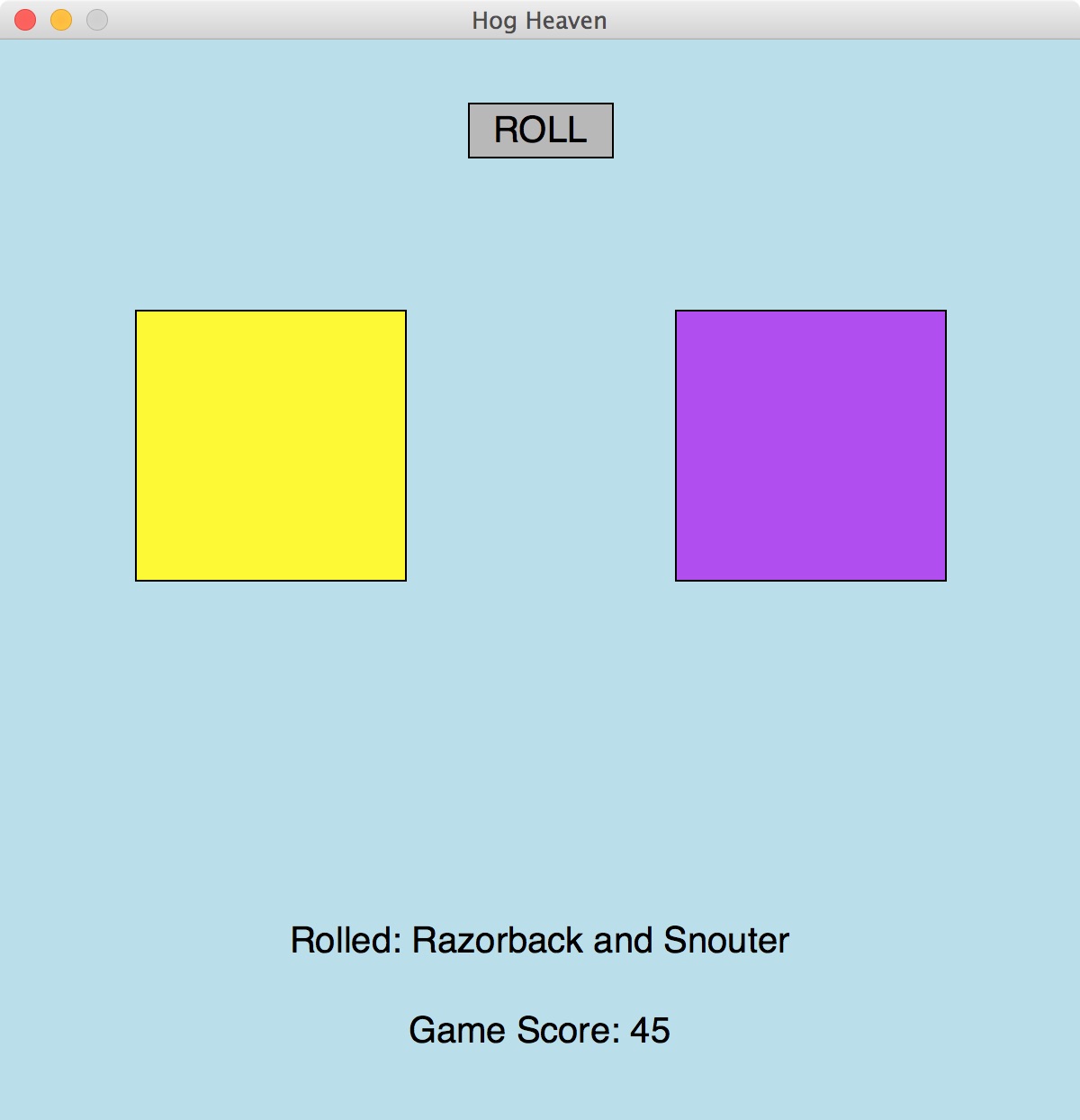
Final Code
When the total score exceeds 100 points, draw a big, colorful congratulatory message on the screen. Your congratulatory message should not block the text at the bottom of the screen or the color rectangles representing the roll. Wait for the user to click anywhere in the window, and then close the graphical window and the program. The graphical window should close cleanly and not freeze. The functionality of drawing the congratulation message should be in its own function that you design and document.
There is no demo for your final code.
Samples
- Sample 6 (Window)
- Sample 7
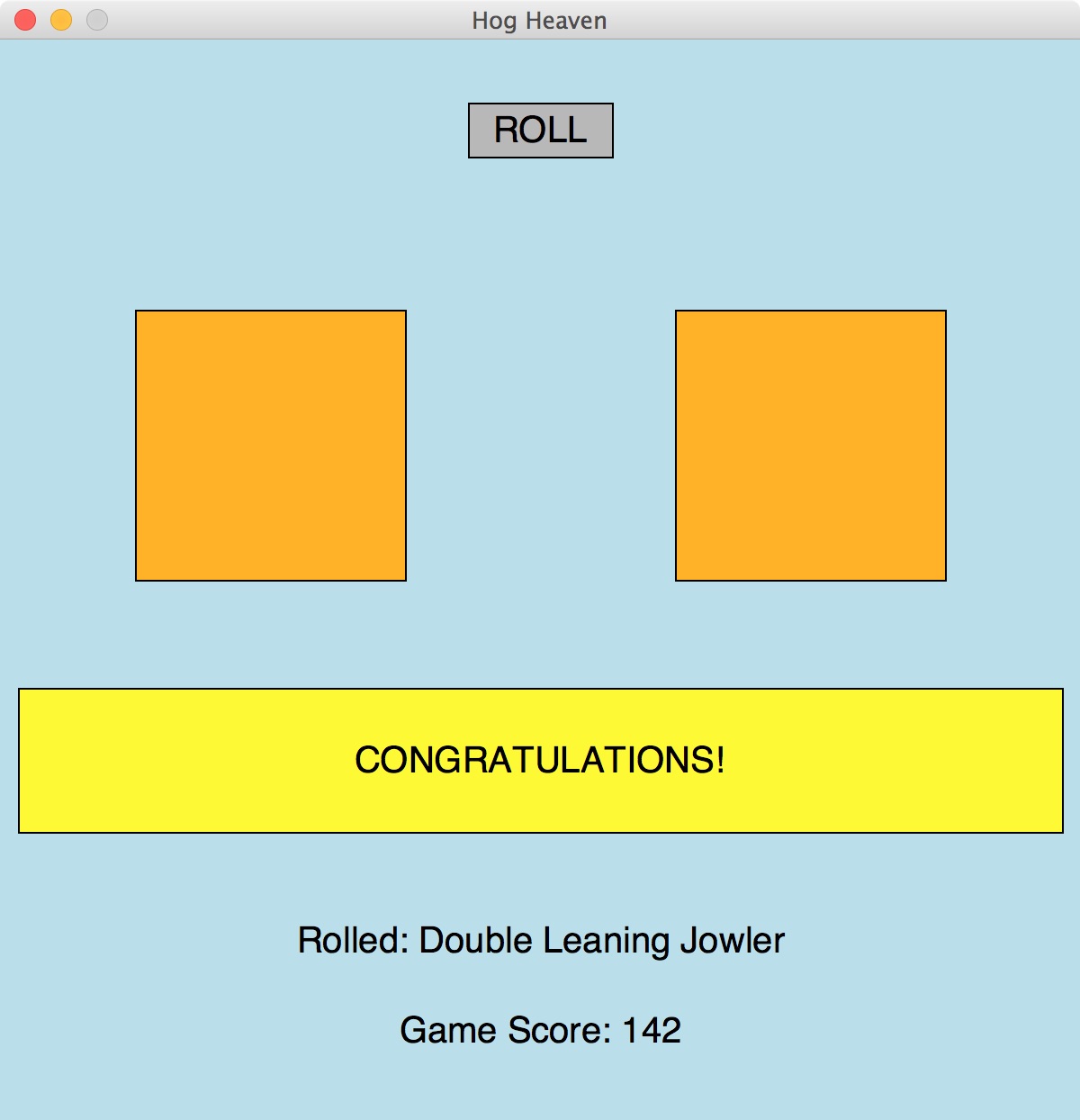
You can test your program by modifying the roll()
function to produce specific rolls. When you give the roll()
function a numeric argument, it will run through a set of pre-assigned rolls. The samples below show that behavior for the numbers 1--4, so you can test your code.
Using roll(1)
:
Box 1 | Box 2 | Name | Total score |
---|---|---|---|
red | red | Sider | 1 |
red | blue | Pig Out | 1 |
red | yellow | Razorback | 6 |
red | green | Trotter | 11 |
red | purple | Snouter | 21 |
red | orange | Leaning Jowler | 36 |
blue | red | Pig Out | 36 |
blue | blue | Sider | 37 |
blue | yellow | Razorback | 42 |
blue | green | Trotter | 47 |
blue | purple | Snouter | 57 |
blue | orange | Leaning Jowler | 72 |
yellow | red | Razorback | 77 |
yellow | blue | Razorback | 82 |
orange | orange | Double Leaning Jowler | 142 |
Using roll(2)
:
Box 1 | Box 2 | Name | Total score |
---|---|---|---|
yellow | yellow | Double Razorback | 20 |
yellow | green | Mixed Combo (Razorback + Trotter) | 30 |
yellow | purple | Mixed Combo (Razorback + Snouter) | 45 |
yellow | orange | Mixed Combo (Razorback + Leaning Jowler) | 65 |
green | red | Trotter | 70 |
green | blue | Trotter | 75 |
green | yellow | Mixed Combo (Trotter + Razorback) | 85 |
green | green | Double Trotter | 105 |
Using roll(3)
:
Box 1 | Box 2 | Name | Total score |
---|---|---|---|
green | purple | Mixed Combo (Trotter + Snouter) | 15 |
green | orange | Mixed Combo (Trotter + Leaning Jowler) | 35 |
purple | red | Snouter | 45 |
purple | blue | Snouter | 55 |
purple | yellow | Mixed Combo (Snouter + Razorback) | 70 |
purple | green | Mixed Combo (Snouter + Trotter) | 85 |
purple | purple | Double Snouter | 125 |
Using roll(4)
:
Box 1 | Box 2 | Name | Total score |
---|---|---|---|
purple | orange | Mixed Combo (Snouter + Leaning Jowler) | 25 |
orange | red | Leaning Jowler | 40 |
orange | blue | Leaning Jowler | 55 |
orange | yellow | Mixed Combo (Leaning Jowler + Razorback) | 75 |
orange | green | Mixed Combo (Leaning Jowler + Trotter) | 95 |
orange | purple | Mixed Combo (Leaning Jowler + Snouter) | 120 |
Extra Credit
You can get extra credit by extending this specification in creative ways. Trivial customizations (like a different background color) will not be accepted. No matter what, the colors of the squares should not be modified or customized.
Here are some ideas: You can make the congratulatory message flash or blink (+2). You can make the squares bigger and include custom images inside them, as long as their color can be seen (+5). Simply should put all supplementary materials for grading into a zip file with your project files, in the exact organization required to run the entire project. Or, suggest your own ideas to us via Piazza.
You will get no more than 10 points total for extra credit. Be sure you describe any extra-credit work in your docstring, explaining the judgements you made and you must include references justifying these judgements. If you do not describe your extra credit work, it will get ignored.
Grading Rubric
- Checkpoints [20%]
-
Checkpoint demos are each worth 10 points; each is all or nothing.
- Programming Design and Style [25%]
-
In addition to being correct, your program should be easy to understand and well documented. For details, see the rubric below.
- Correctness [55%]
-
The most important part of your grade is the correctness of your final program. Your program will be tested numerous times, using different inputs, to be sure that it meets the specification. You will not get full credit for this unless your output matches the sample output exactly for every case, including capitalization and spacing. Attention to detail will pay off on this assignment. For details, see the rubric below.
Detailed Rubric
Correctness: functional features (55 points)
Theroll()
function will be modified to create a specific series of rolls. Your program will be scored on the basis of its correct behavior under those scenarios.
Metric 1 (5 pts): | The initial screen appears as shown in the sample (see Sample 3). |
Metric 2 (5 pts): | Clicking outside the roll box has no effect. |
Metric 3 (9 pts): | First roll has correctly colored boxes, text message and properly updated score. |
Metric 4 (18 pts): | Subsequent rolls have correctly colored boxes, text message and properly updated score (Sample 4, Sample 5, Sample 7). |
Metric 5 (5 pts): | Congratulatory message appears in the correct scenarios and is the right size, i.e., does not obscure the text of colored boxed (Sample 6) |
Metric 6 (8 pts): | Program closes cleanly after clicking anywhere on the window for the congratulatory message. |
Metric 7 (5 pts): | Program works with a variety of window sizes. |
Correctness: spacing, spelling, grammar, punctuation (5 points)
Your spelling, punctuation, etc. get a separate score: each minor error in spacing, punctuation, or spelling gets a score of 2.5, and each major error gets a score of 5. Here is how the score translates to points on the assignment:
[5] | Score = 0 |
-1 | 0 < Score <= 2.5 |
-2 | 2.5 < Score <= 5 |
-3 | 5 < Score <= 7.5 |
-4 | 7.5 < Score <= 10 |
-5 | Score > 10 |
Programming Design and Style (25 points)
- Docstring (3 points)
- There should be a docstring at the top of your submitted file with the following information:
1 pt. Your name (first and last), the course and the assignment. 2 pts. A brief description of what the program does - Use of functions (9 points)
- Program is broken up into logical, well-defined functions. Functions perform specific, well-defined jobs and have descriptive names. Functions are no more than 50 lines of code.
6 pts. Program must have student-written functions obeying above guidance. 3 pts. Functions are fully documented with a docstring confirming to our docstring conventions from class. - Documentation (6 points)
- Not counting the docstring, your program should contain at least three comments explaining aspects of your code that are potentially tricky for a person reading it to understand. You should assume that the person understands what Python syntax means but may not understand why you are doing what you are doing.
6 pts. You have at least 3 useful comments (2 points each) - Variables (3 points)
-
3 pts. Variables have helpful names that indicate what kind of information they contain. - Algorithm (4 points)
-
2 pts. Your algorithm is straightforward and easy to follow. 2 pts. Your algorithm is reasonably efficient, with no wasted computation or unused variables. - Catchall
- For students using language features that were not covered in class, up to 5 points may be taken off if the principles of programming style are not adhered to when using these features. If you have any questions about what this means, then ask.
Submission
You should submit your final code on Moodle by the deadline. I strongly encourage you to take precautions to make and manage backups while you work on your project, in case something goes wrong either while working or with your submission to Moodle.
Name the file you submit to Moodle yourlastnameP2.py
, substituting your actual last name (in lowercase) for yourlastname.
Late Policies
Project late policies are outlined in the course policies page.
Collaboration Policy
Programming projects must be your own work, and academic misconduct is taken very seriously. You may discuss ideas and approaches with other students and the course staff, but you should work out all details and write up all solutions on your own. The following actions will be penalized as academic dishonesty:
- Copying part or all of another student's assignment
- Copying old or published solutions
- Looking at another student's code or discussing it in great detail. You will be penalized if your program matches another student's program too closely.
- Showing your code or describing your code in great detail to anyone other than the course staff or tutor.
References
This assignment is based on the "Pig" CS assignments by Todd Neller (Gettysburg College).